Integrate game via API
Generate a URL to redirect users to a unique game instance
Use the API to generate a unique game instance. Send users to this URL to play your game and collect data.
Generate a unique game URL
To generate a secure game instance URL for your user to play a game, use the /api/authentication/generatePlayUrl
endpoint and specify the app_id
, secret
, game_id
, the player_id
, num_items
, and callback_url
.
Returns a URL you can use to send a user to play a game in 2bttns.
ID of the app you've created in 2bttns
Secret of the app you've created in 2bttns
ID of the game you want to play in 2bttns
ID of the player you want to play in 2bttns. If the player doesn't already exist, it will be created.
GET /api/authentication/generatePlayURL?app_id=text&secret=text&game_id=text&player_id=text HTTP/1.1
Host:
Authorization: Bearer YOUR_SECRET_TOKEN
Accept: */*
text
Find game_id
in Console
game_id
in ConsoleWe can get the game_id
from our Console by navigating to Games:
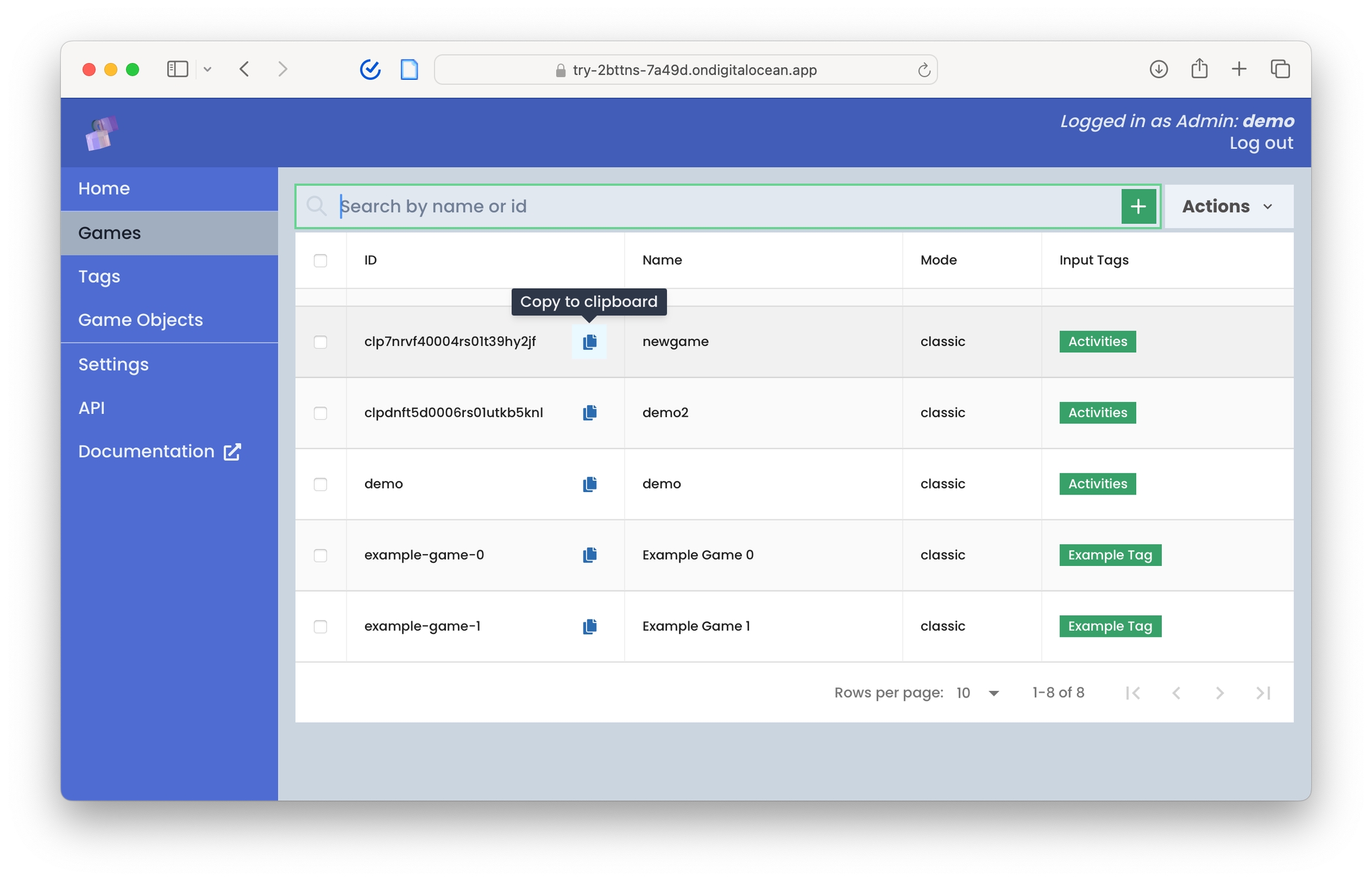
Example
let fetch;
(async () => {
fetch = (await import('node-fetch')).default;
const url = 'http://localhost:3262';
const endpoint = '/api/authentication/generatePlayURL';
const params = {
app_id: "example-app", // Use environment variables as needed
secret: "example-secret-value",
game_id: "booksort",
player_id: "a-user-id",
callback_url: "https://www.example.com",
num_items: 5 // num_items will override Round Length set in your Console
};
try {
const response = await fetch(`${url + endpoint}?app_id=${params.app_id}&secret=${encodeURIComponent(params.secret)}&game_id=${params.game_id}&player_id=${params.player_id}&callback_url=${encodeURIComponent(params.callback_url)}`, {
method: 'GET',
headers: {
'Authorization': `Bearer ${BearerToken}`
}
});
const url = await response.json();
console.log(url);
} catch (error) {
console.error('Error:', error);
}
})();
Last updated
Was this helpful?